Node.js开发环境及应用实例
Node.js 简练,清爽
如何在IDEA中加入默认/内置函数的auto-completion
IDEA node.js 函数的autocomplete
实现了批量编辑文件名含有netsuite的所有文件,添加tags 和 categories
实现了月初的需求:寻求批量分类解决方案
这下面的代码可以灵活拓展,应用在不同的批量修改编辑磁盘目录下的文件内容。
Node.js源代码如下:
1 |
|
Sublime Text 编辑器 打开文件夹目录
Sublime Text 编辑器 打开文件夹目录,它便会加载这个文件夹下的所有文件,(用这种方式更新文章,非常便捷)
然后使用菜单“查找 》 替换”来搜索特定的特征的字符串.
搜索内容,也是支持正则表达
Node.js Dev 步骤
Git clone
- 用IDE编辑器(比如:WebStorm)git clone下来
打开IDE,Terminal Tab会自动切换到clone下来的当前nodejs目录
- npm install
- 如遇到版本问题(比如本机运行环境的版本太低)就去下载安装新版,或者直接把node可执行文件放到/usr/local/bin目录下(MAC环境,我放了四个文件版本就升级了:node,npm,npx,corepack)
- npm run dev
- 如遇到问题,可能需要安装特定的包,比如:npx patch-package
- [可选]npx vite build
- npm install
可直接编辑Node JS代码
网络环境的情况下npm命令前面可能需要爬墙:
1
HTTPS_PROXY="http://127.0.0.1:7890"
调试
1
2
3
4
5
6On MacOS or Linux, run the app with this command:
DEBUG=myapp:* npm start
On Windows Command Prompt, use this command:
set DEBUG=myapp:* & npm start
Node.js Build 步骤
编译到当前项目的dist目录中: npm run build
详细步骤见
Docker编译
docker build -t ipserver .
Node.js的Docker容器
目前完还不会打一整个docker的包,先搞一个Node.js的Docker容器,方便管理
1 | version: "3" |
本小节思路来源:Mac上安装Node.js的Docker容器
Node.js的Docker Deployment步骤
- docker exec -it nodejs bash
- [可选步骤,仅适用于升级发布]
- ps -falx | head -1; ps -falx | grep ‘npm|node’
- kill -9 「node进程id,PPID值」
- node /home/app/blogsearch/bin/www &
- 在命令后面添加一个 &,让node运行在后台;回车以后,还有机会输入其他命令
- nohup node /home/app/blogsearch/bin/www &
- 回车以后,还有机会输入其他命令; 退出 docker exec -it 里面的bash后,程序还在后台运行
疑问:如何让这个node常驻在后台执行,意思是:当按键control+c退出当前docker exec -it nodejs bash时,不要退出这个进程。
已上线2.0版本
实测:Adding full text Search via FlexSearch to a Blog
起步:https://expressjs.com/en/starter/installing.html
1 | mkdir blogsearch |
1 |
|
思路来源:Adding full text Search via FlexSearch to a Blog
## 本地node程序的测试备注: 本地node程序的测试方式为:
- 在WebStorm > Terminal中运行: npm start
- 打开浏览器访问: localhost:3000
- 编辑修改的jade模版会实时展现在本地网页localhost:3000中
- app程序的修改部分, 需要重新启动/运行npm start
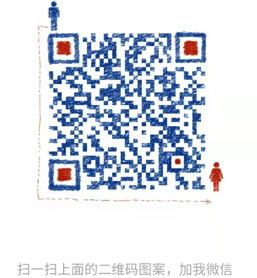